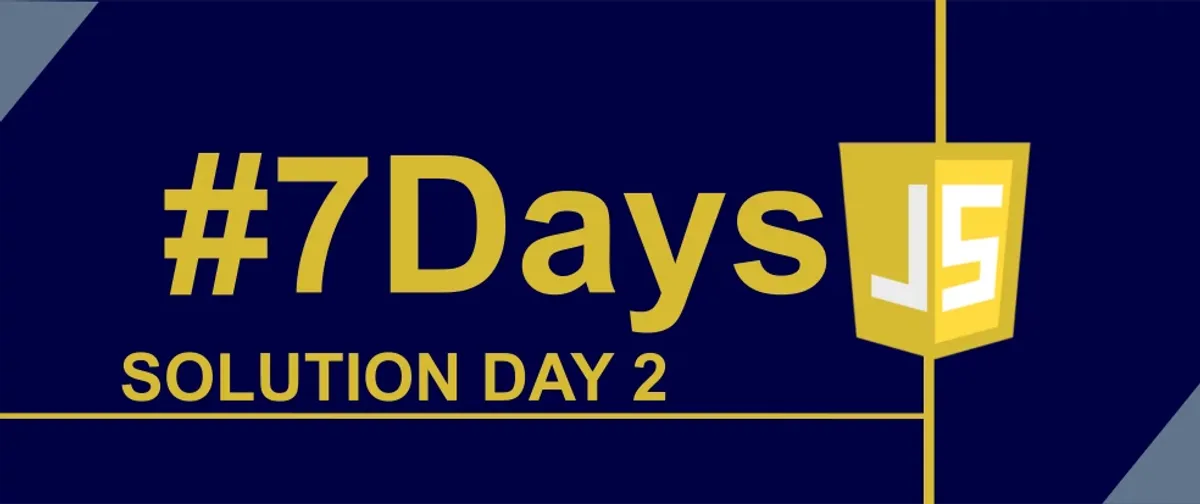
Solution to Day 2 of 7 Days of JS
- JavaScript
- Challenge
- Lautaro Lobo
- 30 Nov, 2019
Let’s see. First of all a solution using a while loop:
function sumboth(array) \{
let count = array.length;
let i = 0, even = 0, odd = 0;
while (i < count) \{
array[i] % 2 == 0 ? even += array[i] : odd += array[i];
i++;
}
return \{even, odd};
}
console.log(sumboth([3,1,6,2]))
And as a second choice, a solution using a for loop:
function sumboth2(array) \{
let count = array.length;
let even = 0, odd = 0;
for (let i = 0; i < count; i++) \{
array[i] % 2 == 0 ? even += array[i] : odd += array[i];
}
return \{even, odd};
}
console.log(sumboth2([3,1,4,2]))
I’ve used the conditional (ternary) operator:
condition ? inCaseIsTrue : inCaseIsFalse;
You can read more about it here.
Any questions? Leave a comment!
See you in the next challenge!