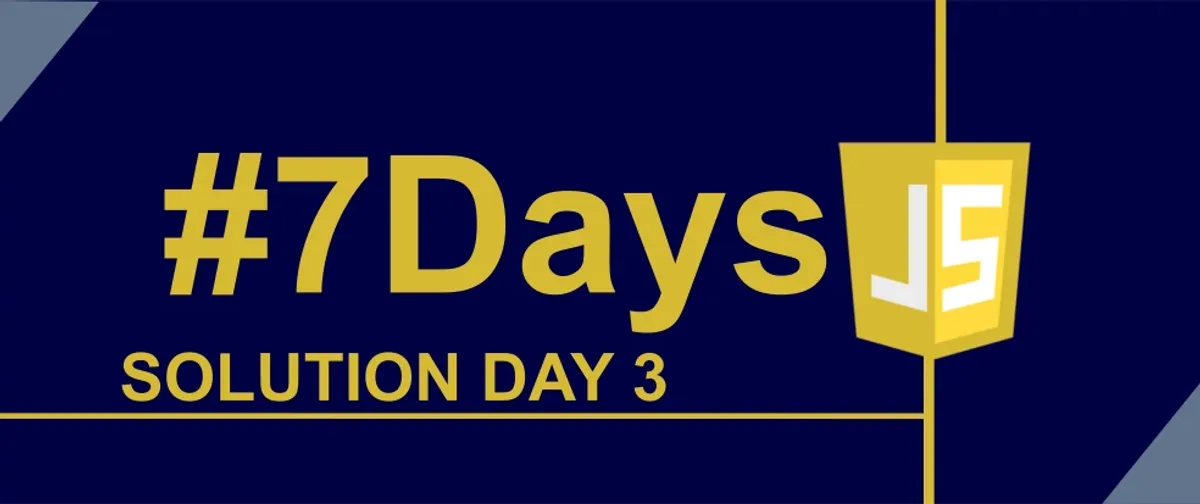
Solution to Day 3 of 7 Days of JS
- JavaScript
- Challenge
- Lautaro Lobo
- 01 Dec, 2019
I wrote first the solution using a while loop:
function sign(array) \{
let count = array.length;
let i = 0, positives = 0, negatives = 0, zeros = 0;
while (i < count) \{
if (array[i] === 0) \{
zeros += 1;
} if (array[i] > 0)\{
positives += 1;
} if (array[i] < 0)\{
negatives += 1;
}
i++;
}
return \{zeros, positives, negatives};
}
console.log(sign([-3,0,6,2]))
And it works! Then, I wrote the same but with a for loop:
function sign(array) \{
let count = array.length;
let positives = 0, negatives = 0, zeros = 0;
for (let i = 0; i < count; i++) \{
if (array[i] === 0) \{
zeros += 1;
} if (array[i] > 0)\{
positives += 1;
} if (array[i] < 0)\{
negatives += 1;
}
}
return \{zeros, positives, negatives};
}
console.log(sign([3,-1,0,-2]))
You may want to write this function using the Math.sign()
method. You can read about it here.
Do you have a different solution? Share it in the comments!