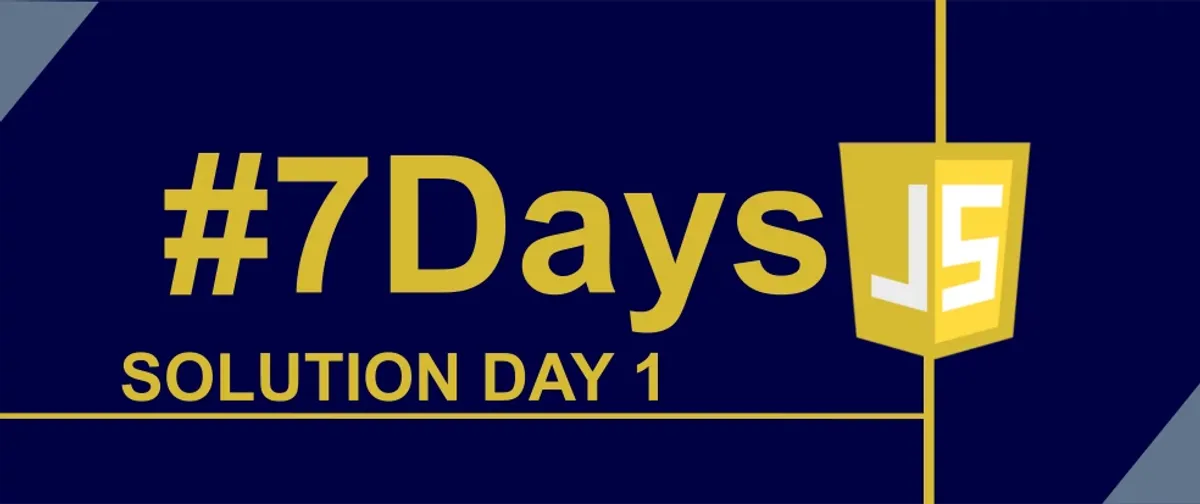
Solution to Day 1 of 7 Days of JS
- JavaScript
- Challenge
- Lautaro Lobo
- 29 Nov, 2019
First of all, we have a functional solution, not the best solution (it may take some time to run with large numbers), but a solution at least:
function factorial(n)
\{
if (n==0 || n==1)\{
return 1;
}
else \{
return n * factorial( n-1 );
}
}
console.log(factorial(5))
The good thing is that is pretty easy to read.
Now, a better solution would be something like this:
function factorial(n)\{
let j = 1;
for (let i = 1; i <= n; i++)\{
j = j * i;
}
return j;
}
console.log(factorial(5))
An imperative solution using a for loop. This makes our function run faster.
Now let’s see the average function. First of all, a solution using a for loop:
function average(array) \{
let count = array.length;
if (count == 0)\{
return 0;
} else \{
var sum = 0;
for (var i = 0; i < count; i++) \{
sum = sum + array[i];
}
return sum / count;
}
}
console.log(average([2,4]))
I also made it using the reduce()
method and an arrow function. Something weird came out:
function average(array) \{
let count = array.length;
if (count == 0)\{
return 0;
} else \{
return array.reduce( (a,b) => a + b) / count;
}
}
console.log(average([2,5,2]));
That’s not very readable, so I prefer the first solution, which btw runs faster.
Both solutions have if and else statements, and you probably noticed why: to return 0 when the input is an empty array, and not some weird error, or the good ol’ NaN
.
Any questions? Leave a comment!